Unlike most template syntaxes, with Razor you do not need to interrupt your coding to explicitly denote the start and end of server blocks within your HTML. The Razor parser is smart enough to infer this from your code. This enables a compact and expressive syntax which is clean, fast and fun to type.
You can learn more about Razor from some of the blog posts I’ve done about it over the last last 9 months:
- Introducing Razor
- New @model keyword in Razor
- Layouts with Razor
- Server-Side Comments with Razor
- Razor’s @: and <text> syntax
- Implicit and Explicit code nuggets with Razor
- Layouts and Sections with Razor
Simple @helper method scenario
The @helper syntax within Razor enables you to easily create re-usable helper methods that can encapsulate output functionality within your view templates. They enable better code reuse, and can also facilitate more readable code. Let’s look at a super-simple scenario of how the @helper syntax can be used.Code before we define a @helper method
Let’s look at a simple product listing scenario where we list product details, and output either the price of the product – or the word “FREE!” if the item doesn’t cost anything:

The above code is fairly straight-forward, and Razor’s syntax makes it easy to integrate server-side C# code within the HTML.
One place that is a little messy, though, is the if/else logic for the price. We will likely output prices elsewhere within the site (or within the same page), and duplicating the above logic everywhere would be error-prone and hard to maintain. Scenarios like this are prime candidates to extract and refactor into helper methods using the @helper syntax.
Refactoring the above sample using the @helper syntax
Let’s extract the price output logic, and encapsulate it within a helper method that we’ll name “DisplayPrice”. We can do this by re-writing the sample to the code below:

We’ve used the @helper syntax above to define a reusable helper method named “DisplayPrice”. Just like a standard C#/VB method, it can contain any number of arguments (you can also define the arguments to be either nullable or optional). Unlike standard C#/VB methods, though, @helper methods can contain both content and code, and support the full Razor syntax within them – which makes it really easy to define and encapsulate rendering/formatting helper methods:
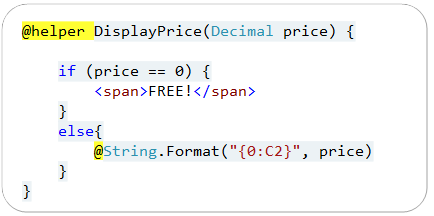
You can invoke @helper methods just like you would a standard C# or VB method:
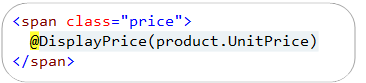
Visual Studio will provide code intellisense when invoking the method:
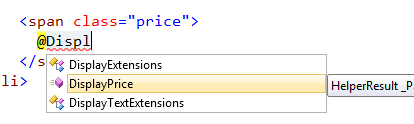
Reusing @helpers across multiple views
In the example above, we defined our @helper method within the same view template as the code that called it. Alternatively, we can define the @helper method outside of our view template, and enable it to be re-used across all of the view templates in our project.We can accomplish this by saving our @helper methods within .cshtml/.vbhtml files that are placed within a \App_Code directory that you create at the root of a project. For example, below I created a “ScottGu.cshtml” file within the \App_Code folder, and defined two separate helper methods within the file (you can have any number of helper methods within each file):
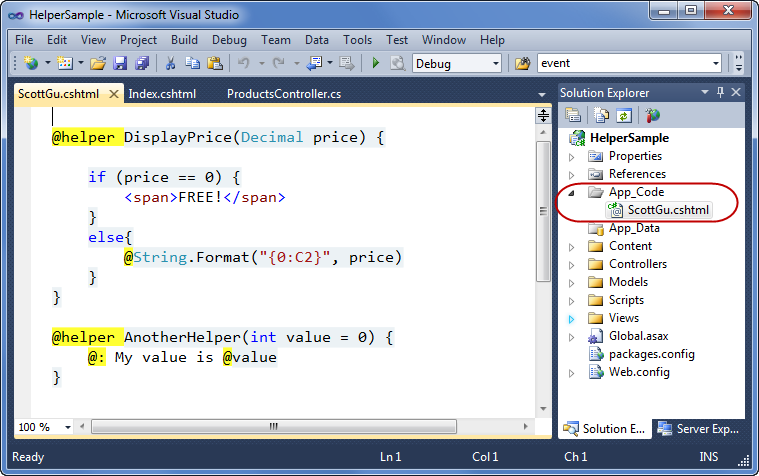
Once our helpers are defined at the app level, we can use them within any view template of our application.
The ScottGu.cshtml template in the \App_Code folder above will logically compile down to a class called “ScottGu” with static members of “DisplayPrice” and “AnotherHelper” within it. We can re-write our previous sample to call it using the code below:

Visual Studio will provide code intellisense when calling app-level helpers like this:

May 15th Update: One issue that a few people have pointed out is that when a @helper is saved within the \app_code directory, you don’t by default get access to the ASP.NET MVC Html helper methods within it (e.g. Html.ActionLink(), Html.TextBox(), etc). You do get access to the built-in HTML helper methods when they are defined in the same file as your views. This is not supported out of the box, though, when the helpers are within the \app_code directory - we’ll add this in the next release. Paul Stovall has a nice helper class that you can use in the meantime to access and use the built-in Html helper methods within @helper methods you define in the \app_code directory. You can learn how to use it here.
Summary
Razor’s @helper syntax provides a convenient way to encapsulate rendering functionality into helper methods that you can re-use within individual view templates, or across all view templates within a project.You can use this functionality to write code that is even cleaner and more maintainable.
Article Source: http://weblogs.asp.net/scottgu/archive/2011/05/12/asp-net-mvc-3-and-the-helper-syntax-within-razor.aspx
No comments:
Post a Comment